SharePoint Technical Articles for SharePoint Developers and IT Pro
Friday, September 20, 2013
Thursday, June 28, 2012
SharePoint 2013 and Office 2013 application development
In this post will find a bunch of useful links about the new SharePoint 2013 and of course Office 2013 application development (this list is constantly updated with new links and materials)
---------------------------------------------------------------------------------------------------------------------------
Download Microsoft SharePoint Server 2013
http://technet.microsoft.com/en-us/evalcenter/hh973397
---------------------------------------------------------------------------------------------------------------------------
Getting started from here:Download the tools, products, SDK and resources you need to create apps for Office and SharePoint:
http://msdn.microsoft.com/en-US/office/apps/fp123627
Microsoft Office Developer Tools for Visual Studio 2012
All the tools require Visual Studio 2012 RC!!.
Download the Visual Studio 2012 Ultimate RC here: http://go.microsoft.com/fwlink/?linkid=247148
Learn what apps for SharePoint are, why you should build them, and the concepts that are fundamental to building them in SharePoint 2013 Preview.
http://msdn.microsoft.com/en-us/library/office/apps/fp179930(v=office.15)
How to start building apps for Office 2013 and SharePoint 2013:
http://msdn.microsoft.com/en-US/office/apps
Code samples of Apps for Office and SharePoint from MSDN:
http://code.msdn.microsoft.com/officeapps
.NET client API reference for SharePoint 2013
http://msdn.microsoft.com/en-us/library/office/apps/jj193041(v=office.15)
How to Publish apps for Office and SharePoint
http://msdn.microsoft.com/en-us/library/office/apps/jj220037(v=office.15)
Older Links
---------------------------------------------------------------------------------------------------------------------------
SharePoint 15 Technical Preview Interoperability API Documentation
http://www.microsoft.com/en-us/download/details.aspx?id=28768
http://msdn.microsoft.com/en-us/library/office/apps/fp179930(v=office.15)
How to start building apps for Office 2013 and SharePoint 2013:
http://msdn.microsoft.com/en-US/office/apps
Code samples of Apps for Office and SharePoint from MSDN:
http://code.msdn.microsoft.com/officeapps
.NET client API reference for SharePoint 2013
http://msdn.microsoft.com/en-us/library/office/apps/jj193041(v=office.15)
How to Publish apps for Office and SharePoint
http://msdn.microsoft.com/en-us/library/office/apps/jj220037(v=office.15)
Older Links
---------------------------------------------------------------------------------------------------------------------------
SharePoint 15 Technical Preview Interoperability API Documentation
http://www.microsoft.com/en-us/download/details.aspx?id=28768
Saturday, January 28, 2012
SharePoint Visual Web Part with Exchange 2010 Integration
In this article we are going to show you how you can create new visual web part that connect to the Exchange Server and load the current user items. For this demo we will load only Calendar and Tasks items, but you can get all the other Exchange items using the same approach.
Before we start, we need to reference the exchange web service .DLL
Next step is creating new Visual Web Part in Visual Studio - this way it will be easier to maintain the UI later.
In ExchangeWebPart.cs we are going to create few properties we will need later - like Exchange Server Service URL, few configurations to define which items to show, limit the umber of items that will be loaded etc:
After we create all the properties we need, we have created a method that will pass the values of these properties to the actual web part (to the .ascx)
At this stage we have everything we need: visual web part, properties and values that are passed to the actual user control. Let's have a look at the .ASCX file: we are going to load the items in Grid View with 3 columns only: Type of Exchange item,Date and a "View Details" link:
Now come the interesting part. I have defined new DataTable variable on the page that will be used as a DataSource if the Grid View. Will fill the DataTable with items from the Exchange Server. Here is how the method looks that Initialize the table and initialize the Exchange Web Service (in this demo will use the Auto-discover option that will return the current user's items:
Once we create the connection to the Exchange Server, based on the web part properties we will get different Exchange items (in this case Tasks and Calendar items):
And further more - let's show how Bind Calendar Items method looks like - nothing special here. Just insert new row in the datable using the data returned by the FindItems method of the Exchange Service. We added also a little additional logic that display the items Received Time if it is today, or display onlt the Date for older items - this is similar to how the Outlook looks like:
Everything is fine and now we can connect to Exchange, load Calendar items from there.. the last thing we are going to do is to load the item's data if the user click on particular item in the DataView. We are using standard RowCommand for this demo. You can also load the Exchange item in new pop-up window or lightbox, or some AJAX fancy control. It's up to you....
Here is how to get the real data for the item:
The important call is this line:
Appointment appointment = Appointment.Bind(service, currentItemId, new PropertySet(BasePropertySet.FirstClassProperties));
This is because FindItems return objects of type
Microsoft.Exchange.WebServices.Data.Item and based on this Item.ID we need to cast the object to the relevant Exchange item:
I.E. here it is not to Bind the Tasks:
Task task = Task.Bind(service, currentItemId, new PropertySet(BasePropertySet.FirstClassProperties));
Once we bind the items, we can easily access the properties we need:
appointment.Start.ToLongTimeString();
appointment.Subject;
appointment.Body.Text;
This is it... nice simple and clear way to connect to Exchange Server using the Web Service (EWS)
The source code will be provided upon request.
Before we start, we need to reference the exchange web service .DLL
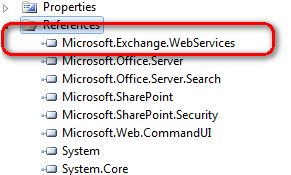
Next step is creating new Visual Web Part in Visual Studio - this way it will be easier to maintain the UI later.
In ExchangeWebPart.cs we are going to create few properties we will need later - like Exchange Server Service URL, few configurations to define which items to show, limit the umber of items that will be loaded etc:
After we create all the properties we need, we have created a method that will pass the values of these properties to the actual web part (to the .ascx)
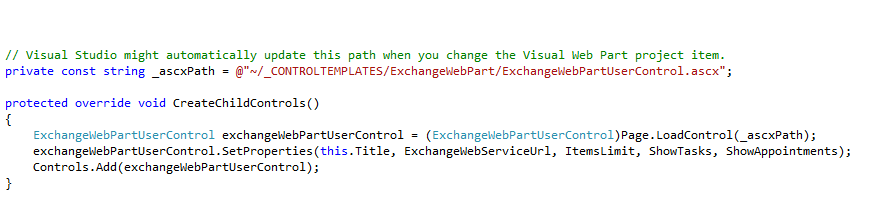
At this stage we have everything we need: visual web part, properties and values that are passed to the actual user control. Let's have a look at the .ASCX file: we are going to load the items in Grid View with 3 columns only: Type of Exchange item,Date and a "View Details" link:
Now come the interesting part. I have defined new DataTable variable on the page that will be used as a DataSource if the Grid View. Will fill the DataTable with items from the Exchange Server. Here is how the method looks that Initialize the table and initialize the Exchange Web Service (in this demo will use the Auto-discover option that will return the current user's items:
Once we create the connection to the Exchange Server, based on the web part properties we will get different Exchange items (in this case Tasks and Calendar items):
Everything is fine and now we can connect to Exchange, load Calendar items from there.. the last thing we are going to do is to load the item's data if the user click on particular item in the DataView. We are using standard RowCommand for this demo. You can also load the Exchange item in new pop-up window or lightbox, or some AJAX fancy control. It's up to you....
Here is how to get the real data for the item:
The important call is this line:
Appointment appointment = Appointment.Bind(service, currentItemId, new PropertySet(BasePropertySet.FirstClassProperties));
This is because FindItems return objects of type
Microsoft.Exchange.WebServices.Data.Item and based on this Item.ID we need to cast the object to the relevant Exchange item:
I.E. here it is not to Bind the Tasks:
Task task = Task.Bind(service, currentItemId, new PropertySet(BasePropertySet.FirstClassProperties));
Once we bind the items, we can easily access the properties we need:
appointment.Start.ToLongTimeString();
appointment.Subject;
appointment.Body.Text;
This is it... nice simple and clear way to connect to Exchange Server using the Web Service (EWS)
The source code will be provided upon request.
Saturday, November 19, 2011
How to call server method with JavaScript and display the result in SharePoint 2010 application page
In this article will try to show you one way you can call server methods using JavaScript from SharePoint page or user control and show the result. For this demonstration I will use simple case: display details about the current logged user. We will load the details from SharePoint and display them in a pop-up (lightbox).
There few components we need to develop in order to make this works:
Step 1: Create simple page (deployed to _layouts). This page will be simple enough and have only 2 elements:
- JavaScript file that contain the javascript finctions
- user control that will display the result
The page "ContractInfo.aspx" is simple and looks like this. Just 1 user control and 2 divs that will display the user profile or error message
Step 2: JavaScript file that will call some of the server side method
JS function looks absolutely normal - nothing special:
function ShowDialog(userId) {
$('#profile-details').show();
try {
//load data
CallServer(userId);
}
catch (err) {
$('#error-details-panel').show();
return false;
}
}
The important call is CallServer(userId). See step 3 for more details
Step 3: Update our .aspx page to inherit ICallbackEventHandler interface.
public partial class ContractDetails: ICallbackEventHandler{ .. }
There are 2 methods we need to implement:
string GetCallbackResult();
void RaiseCallbackEvent(string eventArgument);
Also we need to register the JavaScript function CallServer(userId) using the ClientScriptManager. For this demo I just put it in the PageLoad method.So the entire implementation of the ContractInfo.aspx.cs looks like this:
Step 4: JSON serializer that will return the data to the page in JSON format
Now, after we defined the GetCallbackResult() this method will actually take the user profile details using the provider developed by us. As it was just created for this demo, there is nothing fancy about it - just load the user data from the Active Directory. The important key here is the JSON serializer - see userInfo.ToJSON();
This method will looks like this:
public string ToJSON()
{
JavaScriptSerializer serializer = new JavaScriptSerializer();
string json = serializer.Serialize(this);
return json;
}
This will basically get the UserInfo object and turn it into JSON format, so the page can read it when we pass it back to the page. Of course it is possible to return some XML also or whatever is easier for you.
Step 5: Create custom user control (deployed in ControlTemplates) that will display the results returned by using JSON. This user control is actually just a simple .ascx without any code behind. It will be used only to display the data returned by the server side code.
Here is shown how this ProfileDetails.ascx looks like:
If you look at the PageLoad of our initial ContactInfo.aspx you will note the callback event reference:
String cbReference = clientScript.GetCallbackEventReference(this, "arg", "ReceiveServerData", "context");
ReceiveServerData is actually JavaScript function that read the JSON response and show the data.
function ReceiveServerData(result) {
var myProfileData = null;
try { myProfileData = eval('(' + result + ')'); } catch (err) { }
$('#firstName')[0].innerHTML = (myProfileData.FirstName == null) ? "" : myProfileData.FirstName;
$('#lastName')[0].innerHTML = (myProfileData.LastName == null) ? "" : myProfileData.LastName;
$('#jobTitle')[0].innerHTML = (myProfileData.JobTitle == null) ? "" : myProfileData.JobTitle;
$('#businessPhone')[0].innerHTML = (myProfileData.BusinessPhone == null) ? "" : myProfileData.BusinessPhone;
var picturePath = "/_layouts/YTT/Handlers/GetImage.ashx?acct=" + myProfileData.LoginName;
$('#contactPhoto').attr("src", picturePath);
}
To summarize it quickly e have the following scenario: aspx page that implement ICallbackEventHandler. This allows you to execute server code from JavaScript function, serialize the data to JSON and return it back to the page using another JavaScript function that load the data into custom user control.
There few components we need to develop in order to make this works:
- web part/layouts page/user control
- JavaScript file that will call some of the server side method
- Update the page to implement ICallbackEventHandler
- JSON serializer that will return the data to the page in JSON format
- custom user control deployed in ControlTemplates that will display the result
Step 1: Create simple page (deployed to _layouts). This page will be simple enough and have only 2 elements:
- JavaScript file that contain the javascript finctions
- user control that will display the result
The page "ContractInfo.aspx" is simple and looks like this. Just 1 user control and 2 divs that will display the user profile or error message
Step 2: JavaScript file that will call some of the server side method
JS function looks absolutely normal - nothing special:
function ShowDialog(userId) {
$('#profile-details').show();
try {
//load data
CallServer(userId);
}
catch (err) {
$('#error-details-panel').show();
return false;
}
}
The important call is CallServer(userId). See step 3 for more details
Step 3: Update our .aspx page to inherit ICallbackEventHandler interface.
public partial class ContractDetails: ICallbackEventHandler{ .. }
There are 2 methods we need to implement:
string GetCallbackResult();
void RaiseCallbackEvent(string eventArgument);
Also we need to register the JavaScript function CallServer(userId) using the ClientScriptManager. For this demo I just put it in the PageLoad method.So the entire implementation of the ContractInfo.aspx.cs looks like this:
Step 4: JSON serializer that will return the data to the page in JSON format
Now, after we defined the GetCallbackResult() this method will actually take the user profile details using the provider developed by us. As it was just created for this demo, there is nothing fancy about it - just load the user data from the Active Directory. The important key here is the JSON serializer - see userInfo.ToJSON();
This method will looks like this:
public string ToJSON()
{
JavaScriptSerializer serializer = new JavaScriptSerializer();
string json = serializer.Serialize(this);
return json;
}
This will basically get the UserInfo object and turn it into JSON format, so the page can read it when we pass it back to the page. Of course it is possible to return some XML also or whatever is easier for you.
Step 5: Create custom user control (deployed in ControlTemplates) that will display the results returned by using JSON. This user control is actually just a simple .ascx without any code behind. It will be used only to display the data returned by the server side code.
Here is shown how this ProfileDetails.ascx looks like:
If you look at the PageLoad of our initial ContactInfo.aspx you will note the callback event reference:
String cbReference = clientScript.GetCallbackEventReference(this, "arg", "ReceiveServerData", "context");
ReceiveServerData is actually JavaScript function that read the JSON response and show the data.
function ReceiveServerData(result) {
var myProfileData = null;
try { myProfileData = eval('(' + result + ')'); } catch (err) { }
$('#firstName')[0].innerHTML = (myProfileData.FirstName == null) ? "" : myProfileData.FirstName;
$('#lastName')[0].innerHTML = (myProfileData.LastName == null) ? "" : myProfileData.LastName;
$('#jobTitle')[0].innerHTML = (myProfileData.JobTitle == null) ? "" : myProfileData.JobTitle;
$('#businessPhone')[0].innerHTML = (myProfileData.BusinessPhone == null) ? "" : myProfileData.BusinessPhone;
var picturePath = "/_layouts/YTT/Handlers/GetImage.ashx?acct=" + myProfileData.LoginName;
$('#contactPhoto').attr("src", picturePath);
}
To summarize it quickly e have the following scenario: aspx page that implement ICallbackEventHandler. This allows you to execute server code from JavaScript function, serialize the data to JSON and return it back to the page using another JavaScript function that load the data into custom user control.
Thursday, October 6, 2011
Troubleshooting Search Service stuck in "Stopping State" ...
I have recently experienced this issue: search service stuck in "Stopping" state after last crawl.
After trying almost everything I had in mind:
So I had to finally do enforce the security on the server - this way basically we "unlocked" the component that was faulting the provisioning of the search service. This was done by using the following power shell command:
psconfig.exe -cmd secureresources
Do not panic from the output message. It looks a bit scary:
SharePoint Products Configuration Wizard version 14.0.6009.1000. Copyright Microsoft Corporation 2010. All rights reserved.
Performing configuration task 1 of 5
Initializing SharePoint Products upgrade...
Waiting to get a lock to upgrade the farm.
Successfully initialized SharePoint Products upgrade.
Performing configuration task 2 of 5
Initiating the upgrade sequence...
Successfully initiated the upgrade sequence.
Performing configuration task 3 of 5
Securing the SharePoint resources...
* * *
After all of the steps are done, the farm will be online again.
Have in mind that this command also enforced the security on files, folders, and registry keys, but did the magic - our search service was back to it's normal state
After trying almost everything I had in mind:
- restart IIS
- restarting the service from the services.msc
- trying to stop the service from Central Administration "Services in Server"
- manually ending the process in Task Manager
- trying power shell to stop the service using the command "Stsadm -o osearch -action stop"
- trying to unprovision the content sources
'stop' action failed. Additional information: Invalid search service unprovisioning: application 'Search Service Application' still has a ready component 'd9078ea1-0869-46f7-92d6-d89500f03abf-query-0' on server 'SPFARM2010'.
So I had to finally do enforce the security on the server - this way basically we "unlocked" the component that was faulting the provisioning of the search service. This was done by using the following power shell command:
psconfig.exe -cmd secureresources
Do not panic from the output message. It looks a bit scary:
SharePoint Products Configuration Wizard version 14.0.6009.1000. Copyright Microsoft Corporation 2010. All rights reserved.
Performing configuration task 1 of 5
Initializing SharePoint Products upgrade...
Waiting to get a lock to upgrade the farm.
Successfully initialized SharePoint Products upgrade.
Performing configuration task 2 of 5
Initiating the upgrade sequence...
Successfully initiated the upgrade sequence.
Performing configuration task 3 of 5
Securing the SharePoint resources...
* * *
After all of the steps are done, the farm will be online again.
Have in mind that this command also enforced the security on files, folders, and registry keys, but did the magic - our search service was back to it's normal state
Friday, July 15, 2011
Tips & Tricks: IDs of standard out-of-the-box SharePoint fields
Another useful - How To find out the IDs of standard out-of-the-box SharePoint fields (like Title, Created By, Modified, File Size etc):
The best way is to look at the source - the same location where actually SharePoint is reading them. I am always looking at this file when I have to use out of the box fields in my custom content types.
Here is the location of the XML file that describes all fields with their GUIDs, internal names and, type and so on. The file can be found in 14 hive, in the Template -> Features floder, and the name of the feature is "fields". Inside this folder you can find fieldswss.xml that contais all the information we need:
C:\Program Files\Common Files\Microsoft Shared\Web Server Extensions\14\TEMPLATE\FEATURES\fields\fieldswss.xml
Here is an example of the fields description:
<!-- End Shared Among Base\List with Same Internal Name -->
<!-- Shared Among Base\List with Same Internal Name -->
<Field ID="{fa564e0f-0c70-4ab9-b863-0177e6ddd247}"
Name="Title"
SourceID="http://schemas.microsoft.com/sharepoint/v3"
StaticName="Title"
Group="_Hidden"
Type="Text"
DisplayName="$Resources:core,Title;"
Required="TRUE"
FromBaseType="TRUE">
</Field>
<Field ID="{f1e020bc-ba26-443f-bf2f-b68715017bbc}"
Name="WorkflowVersion"
SourceID="http://schemas.microsoft.com/sharepoint/v3"
StaticName="WorkflowVersion"
Group="_Hidden"
ColName="tp_WorkflowVersion"
RowOrdinal="0"
Hidden="TRUE"
ReadOnly="TRUE"
Type="Integer"
DisplayName="$Resources:core,WorkflowVersion;" />
The best way is to look at the source - the same location where actually SharePoint is reading them. I am always looking at this file when I have to use out of the box fields in my custom content types.
Here is the location of the XML file that describes all fields with their GUIDs, internal names and, type and so on. The file can be found in 14 hive, in the Template -> Features floder, and the name of the feature is "fields". Inside this folder you can find fieldswss.xml that contais all the information we need:
C:\Program Files\Common Files\Microsoft Shared\Web Server Extensions\14\TEMPLATE\FEATURES\fields\fieldswss.xml
Here is an example of the fields description:
<!-- End Shared Among Base\List with Same Internal Name -->
<!-- Shared Among Base\List with Same Internal Name -->
<Field ID="{fa564e0f-0c70-4ab9-b863-0177e6ddd247}"
Name="Title"
SourceID="http://schemas.microsoft.com/sharepoint/v3"
StaticName="Title"
Group="_Hidden"
Type="Text"
DisplayName="$Resources:core,Title;"
Required="TRUE"
FromBaseType="TRUE">
</Field>
<Field ID="{f1e020bc-ba26-443f-bf2f-b68715017bbc}"
Name="WorkflowVersion"
SourceID="http://schemas.microsoft.com/sharepoint/v3"
StaticName="WorkflowVersion"
Group="_Hidden"
ColName="tp_WorkflowVersion"
RowOrdinal="0"
Hidden="TRUE"
ReadOnly="TRUE"
Type="Integer"
DisplayName="$Resources:core,WorkflowVersion;" />
Tuesday, July 5, 2011
Tips & Tricks: quick deployment of .DLLs to GAC
Quick Tips & Tricks
In this post I will try to put some of the tips & tricks we are using every day as a SharePoint developers. Hopefully you will find something that will help you and make your life a bit easier
Tip 1 - quick deployment of .DLLs:
One of the most used by me: PS script for quick deployment of DLLs in GAC and recycle the application pools. Every SharePoint developer is doing this at least 20 times per day. Of course we can use the deployment options of Visual Studio but usually when the change is in code behind we want a short way to replace only the current .dll and recycle the application pools with 1 click only (compared to CKSDev tools that will require at least 4 mouse clicks for the same):
Step 1: Create PowerShell script for deployment to GAC and app pool recycling. Let's name it RecycleCoreDLL.ps1 with the following code:
$appPoolName = "intranet.sharepointmasters.eu"
$appPool = get-wmiobject -namespace "root\MicrosoftIISv2" -class "IIsApplicationPool" | Where-Object {$_.Name -eq "W3SVC/APPPOOLS/$appPoolName"}
$appPool.Recycle()
& 'C:\Program Files (x86)\Microsoft SDKs\Windows\v7.0A\Bin\gacutil.exe' /u SharepointMasters.Portal.Core
& 'C:\Program Files (x86)\Microsoft SDKs\Windows\v7.0A\Bin\gacutil.exe' /i C:\Projects\SharepointMasters\SharepointMasters.Portal.Core\bin\Debug\SharepointMasters.Portal.Core.dll
& 'C:\Program Files (x86)\Microsoft SDKs\Windows\v7.0A\Bin\gacutil.exe' /u SharepointMasters.Portal
& 'C:\Program Files (x86)\Microsoft SDKs\Windows\v7.0A\Bin\gacutil.exe' /i C:\Projects\SharepointMasters\SharepointMasters.Portal\bin\Debug\SharepointMasters.Portal.dll
Here it is how it looks like:
All you need to change is the name of Application Pool and name of the assemblies. In this example I have 2 .DLL I want to deploy:
SharepointMasters.Portal.dll
SharepointMasters.Portal.Core.dll
Step 2: create .bat file that will execute the PS script we just created. In my case I created RecycleCore.bat with only 1 line in it:
powershell.exe .\RecycleCoreDLL.ps1
That's it. Now we have 1 click quick deployment
In this post I will try to put some of the tips & tricks we are using every day as a SharePoint developers. Hopefully you will find something that will help you and make your life a bit easier
Tip 1 - quick deployment of .DLLs:
One of the most used by me: PS script for quick deployment of DLLs in GAC and recycle the application pools. Every SharePoint developer is doing this at least 20 times per day. Of course we can use the deployment options of Visual Studio but usually when the change is in code behind we want a short way to replace only the current .dll and recycle the application pools with 1 click only (compared to CKSDev tools that will require at least 4 mouse clicks for the same):
Step 1: Create PowerShell script for deployment to GAC and app pool recycling. Let's name it RecycleCoreDLL.ps1 with the following code:
$appPoolName = "intranet.sharepointmasters.eu"
$appPool = get-wmiobject -namespace "root\MicrosoftIISv2" -class "IIsApplicationPool" | Where-Object {$_.Name -eq "W3SVC/APPPOOLS/$appPoolName"}
$appPool.Recycle()
& 'C:\Program Files (x86)\Microsoft SDKs\Windows\v7.0A\Bin\gacutil.exe' /u SharepointMasters.Portal.Core
& 'C:\Program Files (x86)\Microsoft SDKs\Windows\v7.0A\Bin\gacutil.exe' /i C:\Projects\SharepointMasters\SharepointMasters.Portal.Core\bin\Debug\SharepointMasters.Portal.Core.dll
& 'C:\Program Files (x86)\Microsoft SDKs\Windows\v7.0A\Bin\gacutil.exe' /u SharepointMasters.Portal
& 'C:\Program Files (x86)\Microsoft SDKs\Windows\v7.0A\Bin\gacutil.exe' /i C:\Projects\SharepointMasters\SharepointMasters.Portal\bin\Debug\SharepointMasters.Portal.dll
Here it is how it looks like:
All you need to change is the name of Application Pool and name of the assemblies. In this example I have 2 .DLL I want to deploy:
SharepointMasters.Portal.dll
SharepointMasters.Portal.Core.dll
Step 2: create .bat file that will execute the PS script we just created. In my case I created RecycleCore.bat with only 1 line in it:
powershell.exe .\RecycleCoreDLL.ps1
That's it. Now we have 1 click quick deployment
Subscribe to:
Posts (Atom)